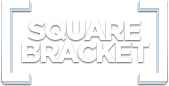
Debugging Like a Pro
I can't tell you how important it is to know how to debug code. So as many of you know, I'm on the youtube making training videos. Most of these videos are not geared towards beginners and assume some level of programming. Time and time again I get hundreds of people asking me
"Whats wrong with my code"
Your failure to debug. Thats whats wrong. Lol. I'm throwing that "lol" in there to be more lighthearted about it. I'm not mad, and not upset that people aren't debugging. I'm able to fix 70% of peoples bugs with the following 2 steps.
1) Paste into TextWrangler.
Literally the syntax highlighting alone will go "HEY LOOK AT ME.. IM A SYNTAX ERROR". If you paste your code into your text editor and its all one color.. Chances are you have a syntax error. Better check that out. Not using an editor that has syntax highlighting? Get one. For mac you have text wrangler, coda, netbeans, text mate. For windows you have eclipse, notepad++, dreamweaver.
2) Firebug's net tab.
So if the code checks out in the text editor, I just open firebug on their site and click the "net" tab. What the net tab will do is simply highlight all the resources in red that failed to connect. Whats a resource? Its a javascript file, a css file or an image. Basically anything you try to link to is a resource. So if your getting "$ is not defined". Chances are your jQuery.js file is not connected. Click the net tab and see confirm that.
"I don't use firefox"
Don't care. Get it, use firebug, deal with it. YES you can use safari and chrome's inspector. But honestly I think they are harder to navigate around.
OK, so thats 70% of the errors fixed. Whew. What about the other 30%? Well 10% of those are bad CSS selectors for your jQuery. I can understand this one though. Sometimes you put a # instead of . and you get confused. The best way to debug these, are to use firebug's console and test your selector out.
So now that we've discussed the most common debugging techniques. Lets talk about more advanced ones.
"I feel like my changes aren't being saved"
Happens all the time. Sometimes you're editing the wrong file, or whatever. There are times when you just have to CTRL A > Delete > CTRL S. Yes, select all, delete, and save. Just to see if your changes are even being made. Chances are - you were editing the wrong file.
"I'm using an MVC but my URLs won't work"
So an MVC is a PHP framework model called "Model View Controller". The basis for almost every MVC out there is the htaccess redirect everything to the index.php file thing. So if your URL's aren't working, either you don't have this file, or its not enabled to work. If your using apache, I made a tutorial on how to fix this. http://www.youtube.com/watch?v=9cLcmX3NcvM
"500 error!"
This means 1 of 2 things. You have a syntax error in your htaccess, or a syntax error in your PHP. So look at your htaccess first, but if you haven't recently made changes to it, its time to get into PHP. First place to look is your error log. This should be logging all your PHP errors. Its normally located in /var/log/apache2/errors.log or phperrors.log. If its not there, you need to enable it (or check the location) in your php.ini file. If you can't do any of that then you need to display your errors. So turn on errors.
ini_set('display_errors',1);
error_reporting(E_ALL);
If you are scared about doing this on a live site (which you should be) Check out this tutorial on how to style your fatal errors so its not THAT huge of a concern (for that 1 minute while you debug it)
http://www.youtube.com/watch?v=a5JyrLnjExQ
Now load your error again, and you should be able to see exactly where it is.
NOTE: Coda (for mac) has a really handy PHP syntax checker as a plugin. I highly recommend it.
"mySQL is giving me the wrong results"
Or you are giving mySQL the wrong query. :D
I suggest you echo out your query in full, paste it into a database app on your desktop and debug it in the console. I use sequelPro for mac, and I think windows has some microsoft something. You can use PHPmyAdmin as well, but I really got away from that a long time ago.
Basically you are just gonna look for the mysql error, and if you don't find one, you can quickly tweak your query to get your results, then look at your code and see why your input wasn't what you expected.
"Cannot access _ property of undefined"
This is your favorite javascript error. The debugging trick with these is to console.log() the main object, then look around in that object in the console. Firebug will print out the object in a nice neat cascading way in order traverse the object and its properties. I use this a ton. This will allow you to see what properties actually exist on that object, and where you might have messed up.
TIP!
The best tip I can give for debugging is this PHP function.
function debug($data,$die=0) {
echo "
<pre>";
var_dump($data);
echo "</pre>";
if($die) die();
}
I use it everywhere. Basically you call debug($myVar); and it prints it out nice and neat for you to read. You can even pass debug($myArray,true); and it will print then kill the script. If your are using and MVC - put this function outside in your base controller and then you can use this without having to do $this->debug().